UH2S2L8——表映射到类
表映射类
- 如果我们要将Lua中的表映射到C#中的类对象中,就声明一个对应的自定义类
其中的成员变量命名要和Lua中表的自定义索引一致
但是它可少可多,无非就是忽略
- 值拷贝 改变实例化对象中的值 不会影响我们Lua中的表
- 支持嵌套
先在Lua脚本声明如下内容
1 2 3 4 5 6 7 8 9
| TestClass = { testInt = 2, testBool = true, testFloat = 1.2, testString = "123", testFun = function() print("123123123") end }
|
再在C#脚本中声明对应的类
注意!在这个类中去声明成员变量的名字一定要和Lua脚本那边的一样,且成员变量必须是公共的, 私有和保护变量没办法赋值
这个自定义类中的变量可以更多也可以更少,如果成员变量比lua中的少,就会忽略它,如果成员变量比lua中的多,即使不会赋值也会忽略
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class CallLuaClass { public int testInt; public bool testBool; public float testFloat; public string testString; public UnityAction testFun;
public void Test() { Debug.Log(testInt); } }
|
这样,我们就可以如调用全局变量那样获取类对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class L7_CallClass : MonoBehaviour { void Start() { LuaManager.Instance.Init(); LuaManager.Instance.DoLuaFile("Main"); CallLuaClass obj = LuaManager.Instance.Global.Get<CallLuaClass>("TestClass"); Debug.Log(obj.testInt); Debug.Log(obj.testBool); Debug.Log(obj.testFloat); Debug.Log(obj.testString); obj.testFun(); obj.Test(); } }
|
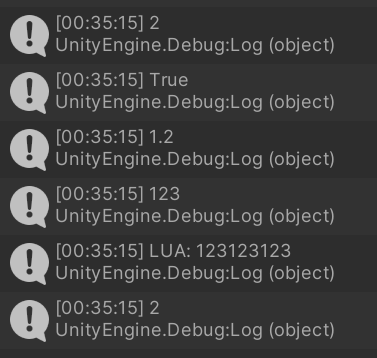
这样获取到的对象仍然是深拷贝,或者说值拷贝,修改该对象无法影响Lua中表的内容
嵌套映射
先在Lua脚本声明如下内容,嵌套一个表
1 2 3 4 5 6 7 8 9 10 11 12
| TestClass = { testInt = 2, testBool = true, testFloat = 1.2, testString = "123", testFun = function() print("123123123") end, testInClass = { testInInt = 5 } } -- 用表模拟的类
|
再在C#脚本中声明对应的类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class CallLuaClass { public int testInt; public bool testBool; public float testFloat; public string testString; public UnityAction testFun; public CallLuaInClass testInClass;
public void Test() { Debug.Log(testInt); } }
public class CallLuaInClass { public int testInInt; }
|
1 2 3 4 5 6 7 8 9 10
| public class L7_CallClass : MonoBehaviour { void Start() { LuaManager.Instance.Init(); LuaManager.Instance.DoLuaFile("Main"); CallLuaClass obj = LuaManager.Instance.Global.Get<CallLuaClass>("TestClass"); Debug.Log(obj.testInClass.testInInt); } }
|
