UFL5-8——UnityWebRequest资源加载模块
前置知识点:
- UnityWebRequest 相关知识 —— Unity网络开发基础
- 泛型 相关知识 —— C#四部曲C#进阶中
- 协同程序 相关知识 —— Unity四部曲之Unity基础中
- 反射中的Type 相关知识 —— C#四部曲C#进阶中
- 委托 相关知识 —— C#四部曲C#进阶中
重要内容回顾
WWW
和 UnityWebRequest
都是Unity提供给我们的简单的访问网页的类,我们可以利用它们来进行数据的上传和下载
除此之外,它们都支持 file://
本地文件传输协议,我们可以利用该协议来异步加载本地文件
特别是在 Android 平台时,我们无法直接通过 C# 中的 File
公共类加载 StreamingAssets
文件夹中的内容
我们需要使用 WWW
或 UnityWebRequest
类来加载
UnityWebRequest资源加载模块我们主要做什么
我们主要封装 UnityWebRequest
当中的
- 获取文本或二进制数据 方法
- 获取纹理数据 方法
- 获取AB包数据 方法
制作 UWQResManager
,让外部使用 UnityWebRequest
加载资源时更加方便
封装UnityWebRequest中的方法
主要将 加载文本、加载文本字节数组、加载纹理、加载AB包 的几个方法n合一,封装在一个泛型异步方法中,方便外部使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| using System; using System.Collections; using UnityEngine; using UnityEngine.Events; using UnityEngine.Networking;
public class UWQResManager : SingletonAutoMono<UWQResManager> { public void LoadRes<T>(string path, UnityAction<T> callBack, UnityAction failCallBack) where T : class { StartCoroutine(ReallyLoadRes<T>(path, callBack, failCallBack)); }
public IEnumerator ReallyLoadRes<T>(string path, UnityAction<T> callBack, UnityAction failCallBack) where T : class { Type type = typeof(T); UnityWebRequest request = null; if (type == typeof(string) || type == typeof(byte[])) request = UnityWebRequest.Get(path); else if (type == typeof(Texture)) request = UnityWebRequestTexture.GetTexture(path); else if (type == typeof(AssetBundle)) request = UnityWebRequestAssetBundle.GetAssetBundle(path); else { failCallBack?.Invoke(); yield break; } yield return request.SendWebRequest(); if (request.result == UnityWebRequest.Result.Success) { if (type == typeof(string)) callBack?.Invoke(request.downloadHandler.text as T); else if (type == typeof(byte[])) callBack?.Invoke(request.downloadHandler.data as T); else if (type == typeof(Texture)) callBack?.Invoke(DownloadHandlerTexture.GetContent(request) as T); else if (type == typeof(AssetBundle)) callBack?.Invoke(DownloadHandlerAssetBundle.GetContent(request) as T); } else failCallBack?.Invoke(); request.Dispose(); } }
|
使用示例
假设要加载StreamingAssets文件夹下的test.txt,UWQResTeach.png,testAB包文件
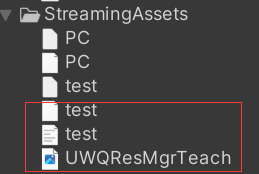
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public class Main : MonoBehaviour { public RawImage img;
void Start() { UWQResManager.Instance.LoadRes<string>($"file://{Application.streamingAssetsPath}/test.txt", (text) => { Debug.Log("加载的文本消息" + text); }, () => { Debug.Log("加载失败"); });
UWQResManager.Instance.LoadRes<byte[]>($"file://{Application.streamingAssetsPath}/test.txt", (bytes) => { Debug.Log("加载的二进制字节数组消息" + bytes.Length); Debug.Log(Encoding.UTF8.GetString(bytes)); }, () => { Debug.Log("加载失败"); }); UWQResManager.Instance.LoadRes<Texture>($"file://{Application.streamingAssetsPath}/UWQResMgrTeach.png", (tex) => { img.texture = tex; }, () => { Debug.Log("加载失败"); }); UWQResManager.Instance.LoadRes<AssetBundle>($"file://{Application.streamingAssetsPath}/test", (ab) => { Debug.Log($"ab包加载成功,名字是:{ab.name}"); }, () => { Debug.Log("加载失败"); }); } }
|
输出: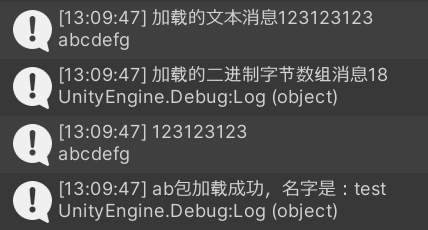