UFL12-2——数字转字符串相关
转字符串数字前补0 的方法
我们在游戏开发中界面显示时,经常可能会有这样的需求,要在界面中显示:
举例:
也就是说想要让某个数字在转换为字符串时,可以指定转换的字符串长度,并且能够在数字前自动的添加0
1 2 3 4 5 6 7 8 9 10 11 12
|
public static string GetNumStr(int value, int len) { return value.ToString($"D{len}"); }
|
使用示例
1 2 3 4 5 6
| void Start() { print(TextUtil.GetNumStr(1, 4)); print(TextUtil.GetNumStr(10, 3)); print(TextUtil.GetNumStr(1000, 4)); }
|
输出:
转字符串保留n位小数 的方法
我们在游戏开发中界面显示时,经常可能会有这样的需求,要在界面中显示,3.1415926
保留两位小数 只显示 3.14
也就是说想要让某个数字在转换为字符串时,可以指定保留小数点后的位数
1 2 3 4 5 6 7 8 9 10 11 12
|
public static string GetDecimalStr(float value, int len) { return value.ToString($"F{len}"); }
|
使用示例
1 2 3 4 5 6
| void Start() { print(TextUtil.GetDecimalStr(3.1415926f, 2)); print(TextUtil.GetDecimalStr(3.1415926f, 4)); print(TextUtil.GetDecimalStr(0.000006f, 2)); }
|
输出: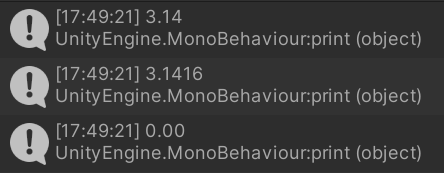
具体代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161
| using System; using System.Collections; using System.Collections.Generic; using System.Text; using UnityEngine; using UnityEngine.Events;
public class TextUtil { #region 字符串拆分相关 public static string[] SplitStr(string str, int type = 1) { if (str == "") return new string[0]; string newStr = str; if (type == 1) { while (newStr.IndexOf(";") != -1) { newStr = newStr.Replace(";", ";"); } return newStr.Split(';'); } else if (type == 2) { while (newStr.IndexOf(",") != -1) { newStr = newStr.Replace(",", ","); } return newStr.Split(','); } else if (type == 3) { return newStr.Split('%'); } else if (type == 4) { while (newStr.IndexOf(":") != -1) { newStr = newStr.Replace(":", ":"); } return newStr.Split(':'); } else if (type == 5) { return newStr.Split(' '); } else if (type == 6) { return newStr.Split('|'); } else if (type == 7) { return newStr.Split('_'); } return new string[0]; }
public static int[] SplitStrToIntArr(string str, int type = 1) { string[] strs = SplitStr(str, type); if (str.Length == 0) return new int[0]; return Array.ConvertAll<string, int>(strs, (str) => { return int.Parse(str); }); }
public static void SplitStrToIntArrTwice(string str, int typeOne, int typeTwo, UnityAction<int, int> callBack) { string[] strs = SplitStr(str, typeOne); if (strs.Length == 0) return; int[] ints; for (int i = 0; i < strs.Length; i++) { ints = SplitStrToIntArr(strs[i], typeTwo); if (ints.Length == 0) continue; callBack.Invoke(ints[0], ints[1]); } }
public static void SplitStrwice(string str, int typeOne, int typeTwo, UnityAction<string, string> callBack) { string[] strs1 = SplitStr(str, typeOne); if (strs1.Length == 0) return; string[] strs2; for (int i = 0; i < strs1.Length; i++) { strs2 = SplitStr(strs1[i], typeTwo); if (strs2.Length == 0) continue; callBack.Invoke(strs2[0], strs2[1]); } } #endregion
#region 数值转字符串相关 public static string GetNumStr(int value, int len) { return value.ToString($"D{len}"); }
public static string GetDecimalStr(float value, int len) { return value.ToString($"F{len}"); } #endregion }
|